Everything You Need To Know About Interpretability in Linear Regression: Net Effects
Linear regression is by far one of the most used machine learning algorithms of all times. But a regular problem in multiple regression is asserting the relative influence of the predictors in the model. Wanna to know an easy way to detemine them on the fly? Go ahead and read the post!
Posted by : Lokesh Kumar ✪ ✪ 13 min read.
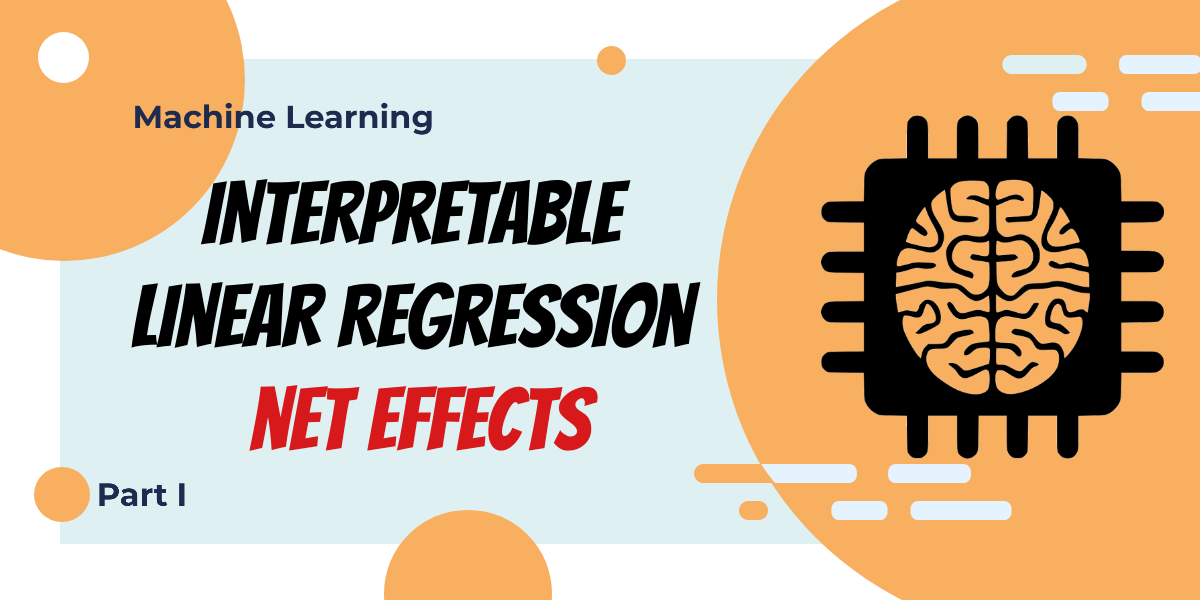
Contents
- Introduction
- Standardized Multiple Regression Model
- Revisiting R-squared for Regression Analysis
- Net Effects
- Problems caused by Multicollinearity
Code used in the blog can be accessed on GitHub
Introduction
Interpretability and explainability in machine learning is a trend right now, because of the demand to understand why and how did the machine learning algorithm predict the way it does. Why would anyone want to know that? Its because if you are taking a million-dollar decision (finance industry) or life-saving decision (medical industry), you cant rely on a model which is a black box. You would need to know what features influenced a machine learning algorithm and analyse whether is it relevant to human intelligence. For example, if you are rejecting a transaction as a fraudulent one, you are required to explain why did you reject and give reasons. You cannot reason out like ‘my deep learning model has an accuracy of xxx% and an f-score of xxx in my test dataset, so I rejected this transaction’. When the machine learning algorithm is predicting that a patient is having cancer, the doctor needs to know why is the machine learning algorithm predicting that way so that doctor can use his experience and come to a conclusion of whether the disease is present or not. By now, you mostly would have understood the importance of this field.
We all know that linear regression is used even today and a widely accepted algorithm as an effective one. Even interpretation of the algorithm is quite straightforward when compared with deep neural networks and other ML algorithms. In this post, we will look into the famous net effect interpretability technique used in linear regression and in the following series of posts, understand the limitations of net effects and develop a more robust incremental net effects metric which performs better even in the case of multicollinearity (correlated input features).
Standardized Multiple Regression Model
Consider we have $n$ predictor variables and target variable . For each data point , these variables assume the form respectively. Now, we can formulate the linear model
\begin{equation} y_i = \beta_0 + \beta_1x_{i1}+\beta_2x_{i2}+…\beta_nx_{in}+\epsilon_i \label{genModel} \end{equation}
which is linear combination of the features, and . can also be rewritten as,
where . Now since , we also know
Our main aim throughout the post will be to engage in answering how each predictor () influences the target variable estimation. We also will try answering the question when our model suffers from multicollinearity (i.e) when predictors are highly correlated.
Lets take we have data samples and we write the above formulation in matrix form,
Let be the estimate of obtained by least squares estimation,
Can you think of any problem here? What if there are some variables in which are very small relative to others (orders of magnitude separation)? What if some features in are correlated with other variables? This will cause ill-conditioning of which in turn results in numerical issues during its inversion. More precisely, we might potentially suffer from round off errors in computation. So, we need to reparameterize the regression model into standardized regression model using correlation transform to circumvent this issue.
Correlation Transformation
Correlation transformation is simple modification of usual feature normalization procedure in machine learning. In feature normalization we make sure that each feature is centered (0 mean) and unit standard deviation. Standard feature normalization is given by these equations below,
where are means of columns respectively, and are their respective standard deviations. The correlation transformation requires only one alteration, that is,
The regression model with the correlation transformed variables is called the standardized regression model and is defined formally as,
Note the absence of the intercept term (). Its straight forward to observe that the least squares calculation will always result in intercept term being . How to recover original from the estimated ? Substituting in , we get
and now algebraic manipulations and comparing coefficients from the generalized regression model, we get,
Look below for a well-commented code implementing the correlation transform and standardizing the dataset
def standardize_dataset(X,y):
'''
Parameters
---
X: Unnormalized Predictor data instances
y: Unnormalized target data instances
Returns
---
Standardized X and y which can be used
for other tasks
'''
# X = [x_1, x_2, ... x_n]
# no intercept term
# X = N x n matrix
# y = N dim vector
# concatenating (X,y) to perform columnwise standardization
# dataset = N x (n+1) matrix
dataset=np.c_[X,y]
# Taking mean along axis=0 => mean = N dim vector
mean = dataset.mean(axis=0)
sq_diff = (dataset-mean)**2
sq_diff = sq_diff.sum(axis=0)/(N-1)
# Standard deviation taken along axis=0 (columns)
std = np.sqrt(sq_diff)
# Aplying the Correlation Transform on the dataset
dataset = (dataset-mean)/(std*np.sqrt(N-1))
# If beta is the true coefficients for the original model
# The transformed beta which is the solution for standardized model is
# beta_reg=beta[1:]*std[:-1]/std[-1]
X_norm=dataset[:,:-1]
Y_norm=dataset[:, -1]
return X_norm, Y_norm
Important Note:
For simplicity we refer as (the coefficient in standardized regression model).
Due to advantages in dealing with standardized model, we will assume that the input has underwent a covaraince transformation and the model we assume there after is always the standardized model.
We refer as as . (i.e) we assume standardized inputs from now on.
Just to familiarize you with this change, let me write standardized multiple regression with added noise again below, (again the intercept term is omitted)
The least squares objective squared deviation is,
Minimizing this equation with respect to the parameters of the model (or) projecting the parameter vector on to the column space of (here is matrix of standardized feature variables), we obtain a normal system of equations of the form,
where is the matrix of correlations (between features ), is a vector of correlations of with each feature variable. is the vector of regression coefficients. Solving this linear system, we get the solution,
where is the inverse correlation matrix. The square deviation (Residual Sum of Squares, ) can be represented in matrix form as,
Let’s code this and understand what we are exactly doing,
# Note that these functions are a part of a class StandardizedLinearRegression
# For complete code: https://github.com/tlokeshkumar/interpretable-linear-regression
# Lets define a function set_XY(X, Y) which takes as input the data matrix (X) and
# the target vector (y)
def set_XY(self, X, y):
'''
Parameters
---
X: N x n data matrix
y: N dim vector
Computes the least squares solution and stores the estimated parameters
in self.beta_estimate
'''
self.X=X
self.y=y
# Correlation Matrix of predictor variables
# C_{ij} = r_{ij} = correlation between x_i and x_j
# C_{ii} = 1, if X is standardized
self.C = self.X.T.dot(self.X)
# Correlation vector between target variables (y) and
# predictor variables (x): r_{xy}
self.r = self.X.T.dot(self.y)
# Check for 1x1 dimension
if(len(self.C.shape)):
# C^{-1}r
self.beta_estimate = np.linalg.inv(self.C).dot(self.r)
else:
# (1/C)*r (if C is scalar)
self.beta_estimate = (1/self.C)*self.r
Revisiting R-squared for Regression Analysis ()
Measuring the quality of the fit can be done in many ways. One such metric is to use the residual sum of squares . We can also define a relative metric of performance, called the coefficient of determination or coefficient of multiple determination (). To better appreciate what that is,
Let’s consider a simple model, a constant where the effect of predictor variables are not considered.
This will be our baseline model. So we will try to answer how well does our predictions improve when we consider model which captures linear relationships between predictor variables as opposed to a model which completely disregards such a relationship.
You will immediately realize that solution is just the mean of the target variables (). The residual sum of squares of model is called (total sum of squares).
Relative regression fit quality can be quantified by coefficient of multiple determination which is defined as,
where represents the coefficient of multiple determination, is the residual sum of squares a.k.a (see ). is the total sum of squares which measures the deviation of from its mean . is proportion reduction in squared error in using model instead of choosing a simple intercept model .
Its now clear that as the data is standardized.
# Function to find RSS: Residual Sum of Squares (S^2)
def RSS(self):
'''
Residual sum of squares
'''
# Estimated y
y_pred = self.X.dot(self.beta_estimate)
# difference in prediction to true value
# y_true-y_pred
error=self.y - y_pred
# Squared sum of differences = Residual Sum of Squares
return (error).T.dot(error)
# Function to find TSS: Total Sum of Squares
def TSS(self):
'''
TSS for an intercept model
(Y = \beta_0)
'''
# Squared sum of differences between y and its mean
return (y-y.mean()).T.dot(y-y.mean())
# Function to calculate R^2
def r2(self):
'''
R^2 = 1 - (RSS/TSS)
For standardized models,
R^2 = 1 - RSS
(TSS=1)
'''
return 1 - self.RSS()/self.TSS()
Net Effects
Now coming back to the analysis,
Substituting in we get,
is a bounded entity which always lies between 0 to 1. gives a very important decomposition of , (i,e)
Take a moment and let this result sink in. The entities is called the net effect of the predictor (). This quantity plays an important role in gauging the importance of individual predictors in a multiple linear regression setting. Net effect of a predictor is the total influence that predictor has on the target, considering both its direct effect and indirect effect (via correlation with other predictors).
To get a more clear picture, lets separate out the direct effect and indirect effect from . Substitute , in to get
Expanding the matrix product, we get an alternative way of expressing ,
Ah! now the the direct and indirect influences represented by is clear. From the above equation, , which can be divided into direct and indirect counterparts,
Let’s see the code to get an even more clear picture of whats going on.
def net_effect(self):
'''
Returns a n dim vector of net effect of each predictor variable
self.beta_estimate : The least squares solution for the standardized linear regression
self.r : Correlation vector between target variables (y) and predictor variables (x): r_{xy}
= X^Ty (in standardized multiple regression model)
'''
return self.beta_estimate*self.r
Visualizing net effects of each predictor variables and its corresponding regression coefficients,
Specifications
- Given 10-dimensional feature vector
- Noise follows Gaussian distribution
- For the dataset generation code: Visit my GitHub repo
Now, we will briefly look into the details of the multicollinearity problem in linear regression, and see why people make a huge issue out of it.
Problems caused by Multicollinearity
Multicollinearity arrises when the feature variables (predictor variables) are highly correlated among themselves. Some problems are listed below,
-
Makes the parameter values sensitive. Values vary over a wide range when a small change is made to the model. (like removing a feature, reducing the sample size, etc)
-
Estimated standard deviation of predictor variables becomes large (quantified by Variance Inflation Factor)
-
Signs of regression coefficients can be opposite to pair-wise correlations between target variable and the corresponding feature.
-
Statistical significance of regression coefficients becomes questionable, as regression coefficients need not now, reflect the statistical relation that exists among the predictor variable and the target variable.
-
Multicollinearity has bad effects on the analysis of the influence of individual predictors (/variables) on the target variable.
To understand the problems of multicollinearity better, lets investigate has to say. Multicollinearity can change the sign of to opposite to that of the pairwise correlation , which means . What does this mean in terms of direct and indirect influence interpretation of net effect? As direct influence term in , it essentially means, that indirect influence is and overpowers direct influence. Analysing this with help of ,
Does $NEF_j < 0$ mean predictor must be removed from multiple regression formulation?
NO. We will show later in the post that any additional variable increases the coefficient of multiple determination . means that the definition of net effects as is inadequate and not completely representative of variable’s influence. This calls for the modification of net effects formulation (motivation for incremental net effects)!
As an example, let’s take an example of a severely correlated dataset (synthetically generated, with the same parameters as above) and observe its and parameter coefficients,
In the above graph, you can see that NEF values for some predictors are negative, and since is valid irrespective of the presence of multicollinearity or not, we also notice some predictors having NEF values . So the notion of NEF values capturing influences of individual predictors breaks down.
This calls for a more sophisticated and interesting measure called Incremental Net Effects which takes support from co-operative game theory, which I have also plotted in the above plot. You can notice how incremental net effects can spread the influence effectively among the predictors. Also, it has interesting properties like its always positive (even in the correlated case when net effects become negative) and like net effects, they sum up to .
Want to know how to approach problems involving interpretability in linear regression? Do check out The In-Depth Guide to Interpretability Analysis in Linear Regression!